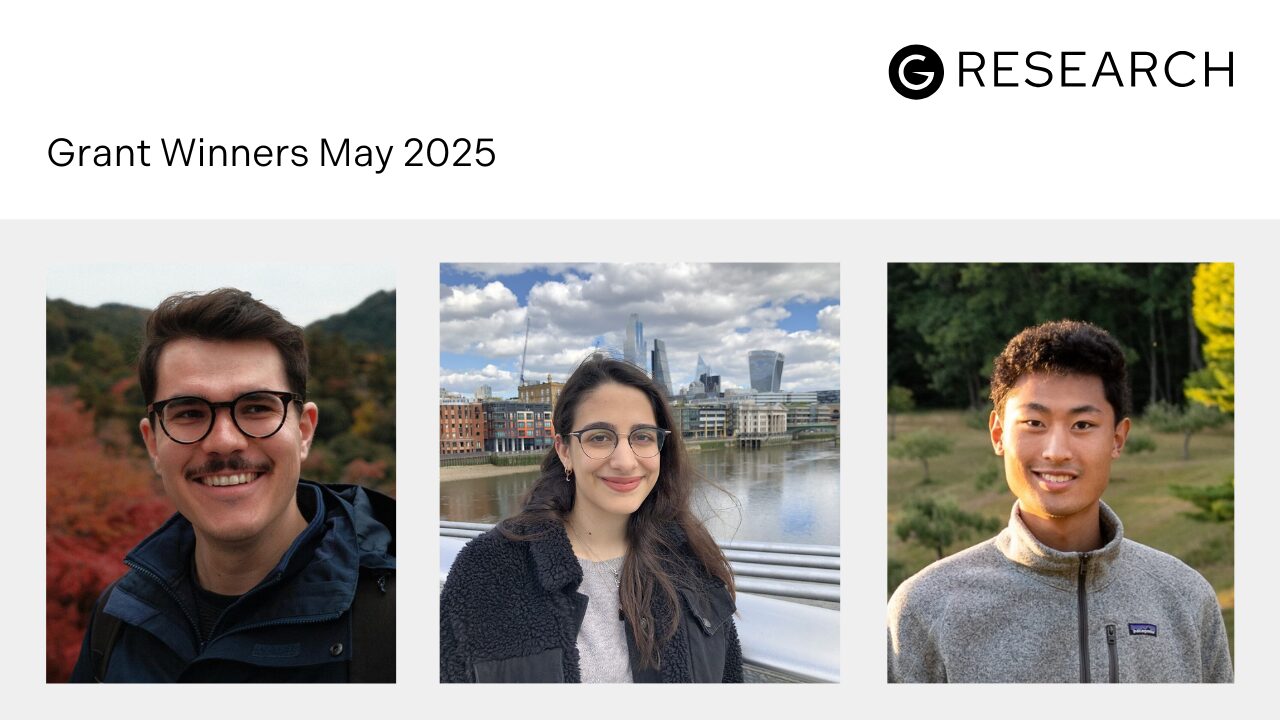
Each month, we provide up to £2,000 in grant money to early career researchers in quantitative disciplines. Hear from our May grant winners.
Read articleEach month, we provide up to £2,000 in grant money to early career researchers in quantitative disciplines. Hear from our May grant winners.
Read articleEvery year, G-Research runs a number of different PhD prizes in Maths and Data Science at universities in the UK, Europe and beyond. We're pleased to announce the winners of this prize, run in conjunction with the University of Warwick.
Read articleEvery year, G-Research runs a number of different PhD prizes in Maths and Data Science at universities in the UK, Europe and beyond. We're pleased to announce the winners of this prize, run in conjunction with the University of Oxford.
Read articleEvery year, G-Research runs a number of different PhD prizes in Maths and Data Science at universities in the UK, Europe and beyond. We're pleased to announce the winners of this prize, run in conjunction with the University of Cambridge.
Read articleEach month, we provide up to £2,000 in grant money to early career researchers in quantitative disciplines. Hear from our April grant winners.
Read articleHear from our Head of Forecasting Engineering on why the term "tech debt" has outlived its usefulness. In this blog, he explores why we should move away from generic labels and instead ask more precise, value-driven questions that lead to meaningful improvements in engineering and business outcomes.
Read articleEach month, we provide up to £2,000 in grant money to early career researchers in quantitative disciplines. Hear from our March grant winners.
Read articleHear from Jay, an Open Source Software Engineer, on tackling technical debt in OpenStack. As technology evolves, outdated code becomes inefficient and harder to maintain. Jay highlights the importance of refactoring legacy systems to keep open-source projects sustainable and future-proof.
Read articleAt G-Research we stay at the cutting edge by prioritising learning and development. That’s why we encourage our people to attend events like SXSW, where they can engage with industry experts and explore new ideas. Hear from two Dallas-based Engineers, as they share their key takeaways from SXSW 2025.
Read articleEach month, we provide up to £2,000 in grant money to early career researchers in quantitative disciplines. Hear from our February grant winners.
Read article